Playing with colors in RGB (red, green, blue) color space is fun and useful. Sometimes using three separate values is difficult, because what you really want to know is what part of the color wheel a shade falls on.
You can use HSV (hue, saturation, value) color space in these cases.
Hue is the color shade on a color wheel. It is often given as a number of degrees on a circle from 0° to 360°. In Python’s pillow library, that is converted to a 0-255 scale. In other places you may find it scaled from 0.0 to 1.0 or 0% to 100%.
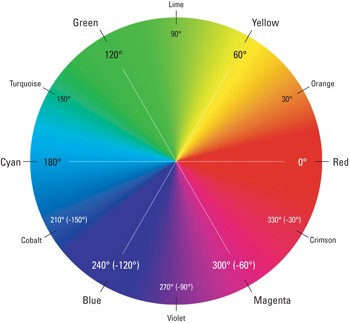
by Deke McClelland, Laurie Ulrich Fuller and Laurie Ulrich
You can see that the primary colors (reg, green, blue) are separated from each other by 120° angles. Halfway between each of these angles, we get each equal combination of two primary colors (yellow, cyan, magenta). Between those angles, we get shades that are dominated by one primary color with a bit of the adjacent primary color.
Saturation (S) is the intensity or vibrancy or purity of a color. A high value of saturation gives an intense color, and a low value gives a color that is mostly gray with just a hint of the color.
Value (V) is the lightness of the color. A low value is close to black, and a high value is close to the maximum lightness of the pure color.
Example Code: making a rainbow gradient using HSV colors
# Create the rainbow gradient image using HSV hue from PIL import Image rainbow = Image.new('HSV', (256, 256)) pix = rainbow.load() for y in range(256): for x in range(256): # changing hue from 0 to 255 to get every color pix[x,y] = (x, 255, 255) # must convert HSV to RGB before saving rgb_rainbow = rainbow.convert('RGB') rgb_rainbow.save('rainbow.png')
In this example, each pixel is set to (x, 255, 255) where x is the x coordinate of the image. The saturation (vibrance of the color) and value (brightness of color) are both set to maximum for every pixel.
This produces the following output image.
Notice that it starts at red (h=0) and cycles through every color until it gets back to red again at h=255.